Retrieving all orders from Binance without specifying a symbol using Python and Binance API
In this article, we will explore how to retrieve all orders from your Binance account without specifying the order symbol using the python-binance
library.
Prerequisites
- Install the
python-binance
library by runningpip install python-binance
in your terminal.
- Make sure you have a valid Binance API token and an active Binance API account.
Code example
import binance.client as client
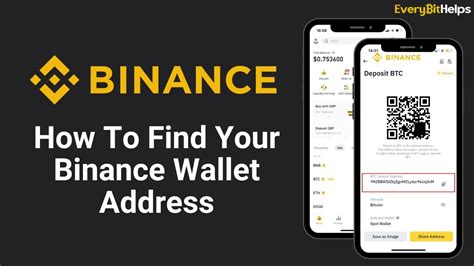
Replace with your actual API token and secret keyapi_token = 'YOUR_API_TOKEN'
secret_key = 'YOUR_SECRET_KEY'
Initialize Binance clientclient_SECRET = clientSecrets.Client(api_token=api_token, secret_key=secret_key)
Define the symbol you want to get all orders for (you can specify any symbol here)symbol = 'BTCUSDT'
Replace with your desired symboltry:
Get all orders without specifying the symbolorders = client.get_all_orders(symbol=symbol, limit=1000)
Get up to 1000 orders for simplicity
Print you the order IDs and quantitiesprint("Order ID\tQuantity")
for i, order in enumerate(orders):
print(f"{order['id']}\t{order['quantity']}")
except Exception as e:
print(f"Error: {e}")
Explanation
In this code example, we first import the client
object from the python-binance
library. Then we initialize the Binance client with your API token and secret key.
We define a symbol for which you want to get all orders (replace it with your desired symbol). The get_all_orders()
function is used to get all orders without specifying the symbol, up to a limit of 1000 orders.
The code contains basic error handling in case an exception occurs while retrieving orders.
Tips and Variations
- To filter orders by specific time periods or account types (e.g. by market cap), you can add additional parameters to the
get_all_orders()
function.
- If you need to retrieve a specific number of orders, use the “limit” parameter instead of 1000. Example: “orders = client.get_all_orders(symbol=symbol, limit=200)”.
- Be aware of Binance’s API rate limits and make sure your code does not exceed these limits.
By following this article, you should now be able to retrieve all orders from your Binance account without specifying the symbol using Python and the “python-binance” library.